Software Lab 11: Just for the Hall of It
Files
This lab description is available as a PDF file here. Code is available here, or by runningathrun 6.01 getFiles
.
In this lab, we will start to look at implementing this behavior on the simulated robot. We will continue this work in Design Lab, and by the end of the week, we will make the robot park itself in a small parking space in the world. We will focus on using probability distributions to model our uncertainty about the robot's motion and observations and using those models in our state estimation framework to estimate the robot's position in a known hallway.
1) Getting Started
You may complete this lab individually or with a partner of your choosing.
You may use any machine that reliably runs soar
.
Get today's files by running
$ athrun 6.01 getFiles
2) Introduction
Last week, we explored Bayesian state estimation in a simple discrete domain by examining how Bayesian updates and transition updates affected our belief about the state of the underlying system. In this lab, we will apply those ideas to estimating the position of the robot in a `soar` simulation. We will start by building a Python framework for general-purpose state estimation, but the bulk of the lab will be spent building probabilistic models of the robot's sensing and motion.We will place the robot at an unknown location in a hallway where the locations of walls are known, and have it drive along a smooth wall on its right using the controller we developed in Design Lab 3. As it drives, we will refine our estimate of the robot's location using sonar readings of the distance to the left wall.
Although the actual robot location and sonar readings have continuous values (in meters), the estimator will represent them with discretized locations (states) and sonar distances (observations), as shown in the figure below, and described by the following:
- States: The robot will only be moving in the
x direction, with real-valued coordinates ranging from
x_min
tox_max
. We will discretize that range intonum_states
equal-sized intervals, and say that when the robot's center is within one of those intervals, then it is in the associated discrete state. The discrete states range from0
tonum_states - 1
, inclusive. - Observations: On each step, the robot will make a distance
observation with its leftmost sonar. The actual readings will be
floating-point numbers between 0 and
sonarMax
. We will discretize this range intonum_observations
equal-sized intervals. The discrete observations range from0
tonum_observations - 1
, inclusive. - Ideal observations: We make a simplifying assumption that
all states with the same distance to the left wall will have the same
observation distribution; to aid in calculating this, we represent
the map using a list called
ideal
. This list is of lengthnum_states
, and it contains, for each state, the distance to the wall on the left, represented as an integer between0
andnum_observations - 1
, inclusive. - Transitions: The robot will move to the right at a constant velocity.
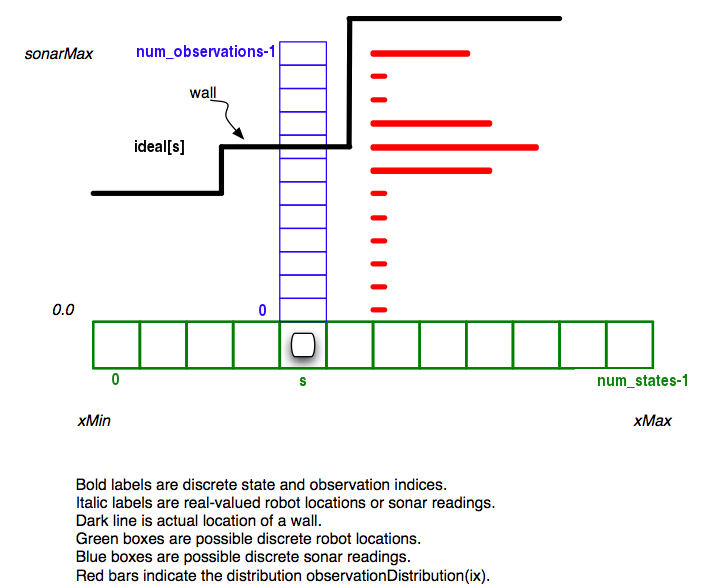
2.1) An Abbreviated Hallway
Consider an abbreviated version of this problem, in which the world consists only of four states, andideal = [1, 8, 8, 1]
. Imagine the robot
starts out thinking it is most likely in state 3, but it isn't sure. So, its
initial belief b_0 is:
|
|
|
|
0.1 | 0.1 | 0.1 | 0.7 |
If its observation model is
def obs_given_loc(loc): if loc == 0 or loc == 3: return DDist({1: .8, 8 : .2}) else: return DDist({1: .2, 8 : .8})
and its transition model is set such that, on each step, the robot moves one room to the right with probability 0.5, and two states to the right with probability 0.5 (assume also that attempting to beyond the right-most room will cause the robot to remain in the right-most room).
For the next three questions, enter your answers as Python lists: $[\Pr(S=0),~\Pr(S=1),~\Pr(S=2),~\Pr(S=3)]$
For example, the initial belief would be entered as `[0.1, 0.1, 0.1, 0.7]`.
2.2) State Estimator
This math can get a little tedious for larger problems, like estimating the
robot's position in a longer hallway. Last week, we implemented the
StateEstimator
class to help with this problem.
At initialization time, StateEstimator
took three arguments:
- an instance of
DDist
representing the original distribution over states, - a function representing the observation model to use (the conditional distribution over observations at time t, given a state at time t), and
- a function representing the transition model to use (the conditional distribution over states at time t+1, given a state at time t).
It also had two important methods:
observe(self, obs)
: updatesself.belief
based on making an observationobs
transition(self)
: updatesself.belief
based on making a transition
Each method updates self.belief
, and does not return anything.
2.3) Simulator
We also used a simulator to help understand how this process worked. If you need a refresher, or if you have trouble with parts of today's lab, you may be interested to run the simulator again to help get a sense of how the state estimation process unfolds.
3) The Brain
Moving forward, we will use this approach to help our 6.01 robot determine its
location as it moves through a world. We will do this by augmenting a
soar
brain (localizeBrain.py
) so that it not only moves the
robot, but keeps a belief over locations the robot might be in. Your job will
be to build distributions that characterize our uncertainty about the robot's
observations and movements.
The file localizeBrain.py
contains code that will:
- Drive the robot at a constant forward velocity from left to right;
- Run the state estimator using the discrete value of the leftmost sonar as input and using models `obs_model` and `trans_model` to describe the domain; and
- Display the belief state and other relevant information while the system is running (the black spike in the top row corresponds to the robot's true location).
Over the course of the rest of the lab, your job will be to supply two critical components:
- the robot's observation model (a conditional distribution over discrete observation values given a discrete state value) and
- the robot's transition model (a conditional distribution over discrete states representing the robot's possible locations on timestep $t$, given a discrete state value representing the robot's location on timestep $t-1$)
Please do not modify other parts of the code.
localizeBrain.py
and make sure they make sense
to you. If not, ask a staff member for help.
The code is set up initially to use naive_obs_model
, which
assumes that we always observe the ideal reading for a location, and
naive_trans_model
, which assumes that the robot moves one
single state per step.
4) Transition Models
Open Soar in the world `gapWorldB.py`. Open the brain `localizeBrain.py`. Run the brain in the world by hitting the `Step` button several times. If an error message is generated, scroll back to the beginning of the red text in the Soar window to read it.
Note that the robot's forward velocity and the timestep length are stored in variables
FORWARD_VELOCITY
and TIMESTEP_LENGTH
, respectively; your code should
make use of these values, rather than hard-coding them.
ideal = [1, 8, 8, 1]
), if the robot starts in state 0,
and we know it moves exactly the width of one state on every timestep, what is the distribution over
states the robot can be in after a transition?
As above, enter your answer as a Python list:
[\Pr(S=0),~\Pr(S=1),~\Pr(S=2),~\Pr(S=3)]
ideal = [1, 8, 8, 1]
), if the
robot starts in state 0, and we know it moves exactly 0.7 times the width of
one state on every timestep, what is the distribution over states the robot can
be in after a transition?
ideal = [1, 8, 8, 1]
), if the
robot starts in state 0, and we know it moves exactly 2.7 times the width of
one state on every timestep, what is the distribution over states the robot can
be in after a transition?Implement an improved transition model that more accurately models the way the
robot moves through the world. Change the variable TRANS_MODEL_TO_USE
to trans_model
, so that the state estimation process uses your new
transition model.
Make sure your fix is robust to the
granularity of the states. Try changing the num_states
variable
on line 52 of localizeBrain.py
. Make sure your localizer works with
num_states = 16
, num_states = 75
, and num_states =
300
when num_observations
is held constant at 30.
Paste your code for trans_model
below.