Python Hall
In this exercise, we will use the DDist
representation developed in
Software Lab 9 to solve a probability problem.
In the Monty Hall problem, you are given the opportunity to select one closed door of three, behind one of which there is a car. The other two doors hide goats. If you pick the door with the car, you win the car.
Once you have selected a door, Monty Hall will open one of the remaining doors, revealing that it does not contain the car. He then asks you whether you would like to change your selection to the other unopened door, or to stick with your original choice. The question is: does it matter if you switch?
The car is equally likely to be behind any door. Assume that your initial strategy is to select a door uniformly at random.
We will consider three discrete random variables, each of which takes values in the set of door numbers \{1,2,3\}:
- C: The number of the door hiding the car,
- S: The number of the door selected by the player,
- H: The number of the door opened by the host
Preliminaries
Answer the following questions about this problem, entering your answers as decimal numbers accurate to within 10^{-3}:
Postliminaries
Assume that the following distributions are defined for you in the box below:
pr_C
is an instance ofDDist
representing the distribution \Pr(C).pr_S
is an instance ofDDist
representing the distribution \Pr(S).pr_H_given_C_S
is a function representing the conditional distribution \Pr(H | C,S).
In the box below, write code to define the following variables by referencing
the above instances of DDist
. While you may find it helpful to
solve for these values by hand to test your code, you should not enter numerical
answers in the box below.
pr_h3_given_s1
should be the probability that the host chooses door 3 given that the player selected door 1.pr_c2_given_h3_s1
should be the probability that the car is behind door 2 given that the player selected door 1 and the host chose door 3.pr_c1_given_h3_s1
should be the probability that the car is behind door 1 given that the player selected door 1 and the host chose door 3.
Given these probabilities, should you make the switch? How much more (or less) likely are you to win the car if you switch? Express your answer as the ratio \frac{\Pr(C=2 | H=3,S=1)}{\Pr(C=1 | H=3,S=1)}.
Or...
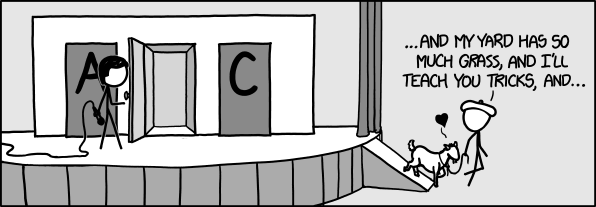
via XKCD