Design Lab 08: Pet Robot
Files
This lab's code distribution is available here, or by running `athrun 6.01 getFiles`.
1) Getting Started
Do this lab with your assigned partner. You and your partner will need a lab laptop (to control a robot). Get today's files from the link above, or by running the following on a 6.01 laptop or Athena machine:$ athrun 6.01 getFiles
Retrieve your circuit board from Software Lab 8. If you did not complete Software Lab 8, you will need to complete it (and get the checkoff) before doing this lab.
2) Pet Robot
We would like to program our robot to follow an infrared LED. To do so, the robot would have to sense the infrared signal, turn to face the source, and then position itself a fixed distance (say 1 foot) from the LED.
The head you built in Software Lab 8 is capable of turning much more quickly than the (massive) robot, so we will construct a two-level control system in which the head turns quickly to face the LED, and the robot body then turns (more slowly) in the direction that the head has turned. This is analogous to your visual system, where your eyes move quickly to track motion and your head turns in the direction of gaze.
2.1) Get a Bearing
Mechanically attach the head to the Lego base plate on the robot. Connect your circuit using a red cable (to the head) and a yellow cable (to the robot) as shown below. **As before, we will power the circuit from the robot, ** so make sure to include a robot connector with pins 2 and 4 connected to the power and ground rails, respectively.
Power on the robot and test your circuit.
Does the head follow motions of the keychain LED? If not, debug your circuit as follows:
- Disconnect the black cable from the motor on the robot head.
- Measure voltages with the multimeter until you find the problem.
Hint: What voltage should drop across the power supply rails? What other known voltages are in the circuit that you can measure? How should the eye voltages change as the light is moved around, and what does that mean for the output of the other op-amps in your circuit?
Our goal is to design and implement a robot
behavior that uses signals from the head to steer the robot toward the keychain
LED. In order to do this, we need a way to access the head's angular position
from SoaR. SoaR can read voltages from the Robot
Connector (see the bottom of this page, or on the back page of the PDF
handout, for complete pinouts for the head
connector and the robot connector) through the io.get_analog_inputs
function,
which takes no inputs and returns a list of
four values representing the voltages on pins 1, 3, 5, and 7, respectively. You
can generate a voltage proportional to the angular position of the head by
connecting the neck potentiometer as a voltage divider, and your circuit generates
voltages proportional to the intensity of light on either eye.
Connect the voltages from the neck potentiometer, the left eye, and the right eye
to pins 1, 3, and 5 of the robot connector, respectively.
Test your connections as follows:
- Remove the black cable from the motor so that you can rotate the robot head manually.
- Open SoaR by running the following command from a terminal: `soar`
- Load and run the `petRobotBrain.py` brain in SoaR.
SoaR will print out the voltages measured on the analog inputs on every step.
Demonstrate to a staff member that the appropriate signals are reaching the brain.
2.2) Total Eclipse of the Heart
Make sure the motor on the robot head is connected (via a black cable) to the "base" of the robot head.
Now modify petRobotBrain.py
to use a proportional controller to make the robot turn left
or right to match the direction of the head, which follows the keychain
LED.
Hint: You can debug the robot behavior by tilting the robot backwards so that the wheels do not touch the ground and watching to see that the behavior is reasonable before unleashing your robot on the world.
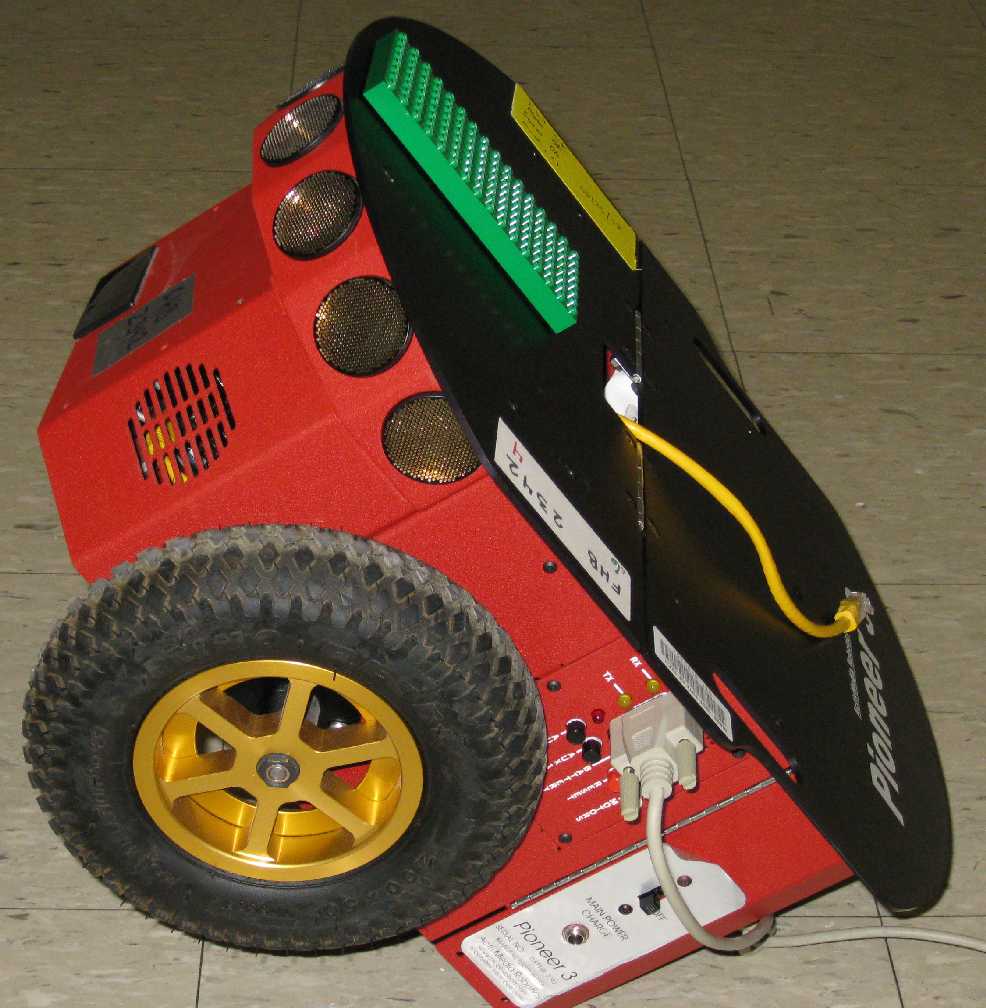
2.3) Keep Your Distance
Rework the brain to make the robot's behavior depend on proximity to the LED. If the LED is off, the robot should stand still in obedience. If the LED is on, the robot should follow the LED, maintaining a distance of approximately 1 foot to the LED. This behavior should not make use of the sonar sensors. Note that it is not necessary to compute an exact distance measurement, so long as you have access to a signal that is roughly proportional to the robot's distance from the light source.
Demonstrate your pet robot's basic behaviors, including facing the LED, approaching the LED, retreating from it, and patiently waiting when the LED is off.
3) Additional Behaviors
Combining the sensory capabilities of the robot head with the existing sensory capabilities of the robot (sonars and odometry) can support a variety of new behaviors. Try any one of the following extensions, or create a new extension of your own.
We have constructed several IR beacons that can be powered from the robot or from the standalone power supplies. If you would like to make use of one of these beacons in your extension, please ask a staff member.
3.1) Robotic Ping Pong
Set up LEDs at opposite ends of a wall. The robot should start by following the wall (as in Design Lab 4), keeping the wall half a meter from its right-facing sonar. When the robot approaches the LED at the end of the road, it should turn 180^\circ and follow the wall in the opposite direction. The robot should then bounce back and forth between the photon bumpers repeatedly.3.2) Robot Ducklings
Team up with another group and work out a scheme to make one robot follow another. The robots could sense each other by attaching an IR beacon to the back of one robot. If you have time, see how long of a convoy you can make!3.3) Junior High Dance
Team up with another group. Attach an IR beacon to the front of each
team's robot, and see what type of sweet dance moves you can create
by adjusting the parameters of each robot's motion and/or by controlling
the IR beacons using the robots' analog outputs (see pinout on attached page, and
the lib601 documentation for soar.io.set_voltage
). But don't let the robots
get too close; the dance chaperones might not approve.
3.4) Remote Control
Program the robot to switch between several behaviors (e.g., wall-following, "dancing" back and forth, spinning in a circle, driving forward, etc) on command. Sending a certain pattern of "blinks" using the IR keychain should cause the robot to switch between these behaviors. Program the robot to listen for these signals, and to change behaviors accordingly.
For an extra challenge, team up with another group, and use one robot's analog output to power an IR beacon to send these signals to the other robot.
3.5) Recording
Program your robot so that, when a light source is nearby, it not only follows the light, but records its motion during that time. When the light is turned off, the robot should "rewind" and then "replay" this motion until it sees another light source.
3.6) For a Good, Clean Feeling, No Matter What
Set up two IR beacons back-to-back to create a 360^\circ IR
source. Adjust the parameters of the robot's motion so that it is able to
orbit this light source. How can you change the parameters of the robot's
motion to cause the robot to orbit at different distances?
You can get the robot to draw its path by setting bread_crumbs=True
in the
on_start
function in petRobotBrain.py
.
3.7) Easily Distracted Robot
Program your robot so that it follows a nearby light source as before, but after a short while following the light, it becomes bored and goes off in search of a different light source to follow. Alternatively, make your robot interested only in light sources that move; if it is following a light source that stays stationary for too long, it should turn away and look for a more interesting light source.
3.8) Going Viral
Program your robot so that, in addition to the behavior from checkoff 2, it turns on an IR beacon on its back when it gets close enough to the light. Team up with another group (or multiple groups) and see whether you can set up a chain reaction to get the robots all to share their light with one another.
3.9) A Light Snack
Construct a "photovore" - a robot that must periodically refuel itself by ingesting photons (from an LED). The robot should do some background task (such as rotating back and forth). However, when the robot gets hungry, it should stop doing that task, and should instead turn toward an LED to catch some rays. If the LED is far away, the robot may have to get closer to satisfy its hunger, before returning to the background task.
3.10) I Am the Keymaster
Set up two IR beacons so that they form a "gateway" wide enough for the robot to pass through. Have the robot spin around, using its light sensor to detect the position of the gate. Once it knows where the gate is, it should drive through it.
3.11) The Life of the Party
Program your robot to spin in place looking for a light source. Once it finds one, it should follow it (using the behavior from checkoff 2). When the light turns off, it should retrace its steps, returning to its original position, where it should spin in place again, waiting for the next light source to follow.
Explain and demonstrate your extended robot behavior to a staff member.
4) Appendix: Circuit Part Pin-outs
4.1) Head Connector
4.2) Robot Connector
4.3) Op-Amp (L272A)
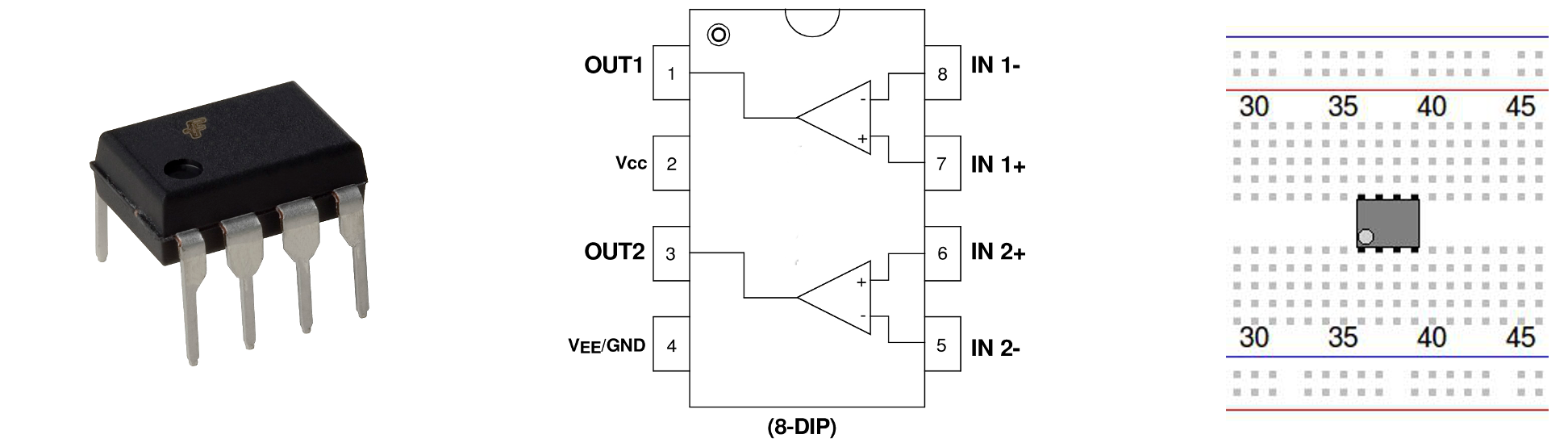