Design Lab 04: We Can Lance If We Want To
Files
This lab description is available as a PDF file here. The code distribution may be downloaded (in zip format) here, or by running `athrun 6.01 getFiles` from an Athena machine or a 6.01 lab laptop.Do this lab with your assigned partner. You and your partner will need a lab laptop to control one of our mobile robots; the parts of the lab not involving the physical robot can be completed on any computer with the 6.01 software installed. Get today's files by running:
$ athrun 6.01 getFiles
1) A New Angle on an Old Problem
Our goal is to program the robot to move along a wall while maintaining a constant distance from the wall. Over the last two labs, we have investigated two control schemes to try to achieve this task. In our first attempt, we tried to correct for tracking errors by steering left in proportion to the difference between the desired and actual distances to the wall. Unfortunately, we _proved_ that no value of the gain `K` would give good performance. Responses were, sadly, oscillatory and divergent for all gains.In Software Lab 4, we began to experiment with proportional angle control and found that by controlling the robot's rotational velocity based off of the error in angle from a desired angle, \theta_i[n], we could quickly and effectively converge towards a line parallel with the wall. The only problem was that we couldn't control the distance from the wall at which the robot converges (see Section 4 of SL04 as a refresher).
Today, we will work to combine ideas from these two controllers to "fix" the wall follower system so that we can program the robot to achieve its goal of driving parallel to a wall at a fixed distance.
To begin, recall the parameters we've been using to describe our robot in
its wallFollowerWorld
, which are illustrated in the diagram below:
1.1) Memories
One of the tasks in software lab was to construct a system to model the way the robot's distance from the wall (D_o) changed in response to its desired angle (\Theta_i).
Draw a block diagram of your system from Software Lab 4. Label the commanded rotational velocity \Omega in your diagram.
1.2) Desires
In our simulations in Software Lab 4, the robot's desired angle was always 0 radians relative to the wall. However, when the robot isn't at its desired distance, we actually want the angle to be something else!
In the figure below, we have marked five different hypothetical locations of the robot in its world. What would be a good angle for the robot at each of these locations? Keep in mind that the robot moves forward with a fixed velocity and wants to move towards its desired path.
Discuss your plot with a staff member. How can this be integrated into what we developed in Software Lab 4?
2) Onward and Rightward
In Software Lab 4, we built a model for how the robot's distance from the wall changed in response to its input angle when it was moving forward with a fixed velocity V and we were setting its angular velocity \omega[n] proportional to its error in angular position \theta_i[n] - \theta_o[n]. Our system had the following form:
In Software Lab, the robot always had a desired angle (\theta_i[n]) of 0 radians. As we saw above, however, this does not always make sense. In actuality, we want to change the desired angle based on the error in distance. Note that, since our model from software lab will work with arbitrary input signals \Theta_i representing the desired angle, we are free to make this change.
One good strategy is to set $\theta_i[n]$ to be proportional to the distance error $d_i[n] - d_o[n]$ so that: $$\theta_i[n] = k_d(d_i[n]-d_o[n])$$In this lab, we will examine whether this controller can solve the problems from the previous labs.
2.1) Wall Follower Model
We can model this new system in the following form:
In this section, we will create a model of this system, first as a block
diagram, and then using the lti
framework we have developed over the last few
weeks.
Draw a block diagram of the entire system below. You may use a box labeled \frac{D_o}{\Theta_i} to represent the system from Software Lab 4 rather than redrawing it.
2.2) Software Model
Now we'll model this system using the framework from last week. A skeleton
file called wallFollowerModel.py
is provided for your work. We have included
functions angle_only_model
and distance_model
, which return instances of
System
representing the two subsystems we explored in Software Lab 4.
angle_only_model
and
distance_model
. How is the function integrator
used?
In wallFollowerModel.py
, write a Python procedure
make_wall_follower_model
that takes the following as input:
k_a
, representing the constant of proportionality between the angular position error and the angular velocity (which we simply called "K" in Software Lab 4)k_d
, representing the constant of proportionality between the distance error and the desired anglestarting_angle
, representing the robot's initial angle relative to the wall (in radians)starting_distance
, representing the robot's initial distance from the wall (in meters)T
, representing the length of one timestep (in seconds)V
, representing the robot's forward velocity (meters per second)
Your function should return an instance of System
that describes the the
behavior of the whole system when the robot is using the proportional angle
controller from Software Lab 4 and setting the desired angle proportional to
the distance error as described above.
Use the functions and methods defined in the lti
module to construct your procedure (see the lib601
documentation for more details).
angle_only_model
and distance_model
within make_wall_follower_model
?
Upload your wallFollowerModel.py
file below.
2.3) Choosing Gains
We can change the robot's behavior by changing $k_a$ and $k_d$. We would like to make the robot converge to its desired distance quickly, but it is not immediately clear how to choose the gains $k_a$ and $k_d$ to accomplish this. Luckily, we can use the poles to predict how the system will behave for particular values of $k_a$ and $k_d$.
To develop a sense for how the poles move as a function of k_a and k_d, we have provided a graphical interface that plots the locations of the poles from the model you defined in the make_wall_follower_model
function with T=0.1, V=0.1.
Run rootLocus.py
to bring up the GUI. The two sliders adjust k_a and k_d and the plot shows the pole locations on the complex plane. Manipulate these sliders to see how the poles move.
Now that you have a sense of how the poles move through the complex plane as the gains are changed, we will write a small bit of code to better understand how to optimize these gains (and to find the optimal values exactly, rather than simply estimating).
Design a function that takes in values of $k_a$ and $k_d$ and returns a single numerical value that tells us something about how quickly the model predicts the robot will converge to its desired location. The $k_a$ and $k_d$ values for which this metric is minimized (or maximized, depending on your metric) should be the "optimum" values of $k_a$ and $k_d$ (that is, the values for which we expect the robot to converge as quickly as possible). Note that in the system we are simulating, $V=0.1 m/s$ and $T=0.1 s$.If the system had a single gain k (as in the system from last week), then we could plot this metric as a function of the gain k, and choose the k for which that metric is optimized. Here, though, we have a system with two gains: k_a and k_d. We can optimize gains for this kind of system by fixing one gain and using a plot to find the value of the other that optimizes our metric.
You can use matplotlib
to generate plots. An example use is shown below:
import matplotlib.pyplot as plt
x_values = list(range(-10,11)) # Note that range only works with integer values
y_values = [i**2 for i in x_values]
plt.plot(x_values, y_values)
plt.title("Plot of y=x^2")
plt.xlabel("x")
plt.ylabel("x^2")
plt.show()
For each value of k_a below, generate a plot of your metric as a function of k_d values (from 0 to 100, with a spacing of at most 0.01), and use this plot to determine the "optimum" value of k_d for that value of k_a. Please label your axes appropriately, and save these plots. Be prepared to discuss these plots (and your choice of metric) with a staff member during a checkoff.
Enter your answers from this section in the table below.
K_a | Best K_d | ||
---|---|---|---|
1.0 |
|
||
2.5 |
|
||
10.0 |
|
||
20.0 |
|
||
3) Analytical
The poles of this system have the following form:
4) Simulating Performance with Soar
Now we will compare our model against some results from the simulated robot in
soar. Open the file wallFollowerBrain.py
in IDLE, where we will implement
our controller. The goal of the on_step
function in the brain is to set the
robot's rotational velocity.
Note: you may find it helpful to first compute the robot's desired angle.
Implement the control scheme we developed in this lab so far by editing
wallFollowerBrain.py
.
Notice that we provide a procedure get_distance_right_and_angle
to calculate
the robot's distance from the wall and angle. The input to the procedure is the
sensed array of eight sonar signals inp.sonars
. The output is a tuple of the
form (distance_right, theta)
, where distance_right
represents the
perpendicular distance to the wall, and theta
represents the angle between
the robot and the wall.
If sonar 6 or 7 is out of range, then we cannot calculate the angle, and the
second component of the output from get_distance_right_and_angle
will be
None
. You should make sure that your soar brain does not produce an error if
and when get_distance_right_and_angle
returns a None
for the angle. One
way to ensure this is to have your brain use the last known value of the angle
(rather than the current angle) when this happens. Of course, that requires
keeping track of the last known value of theta
.
desired_right
has been defined
for you. This contains the robot's desired distance from the wall, 0.4 meters.
You should reference this variable in your brain instead of hard-coding a
desired distance.
Make sure your brain works in the soar
simulator with the
wallFollowerWorld.py
world for each of the gain pairs from above. Save
the plots for each gain pair.
Make sure to "Reload Brain and World" between trials.
The "rotational velocity" graph shows two curves: the blue line represents the
commanded rotational velocity, and the green curve is robot's actual rotational velocity,
as estimated from the robot's theta
values.
Show a staff member your plots from the soar simulations. Compare the simulations to the pole analyses in the previous section. Do the trends that were predicted by the model match those seen in soar? Can you explain the overshoots and oscillations?
5) LEGO Lance, Prince of Mirkwood
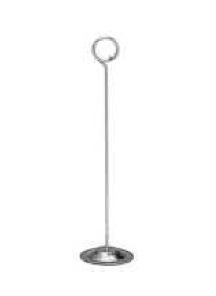
Now we will try to make the robot spear a small target (shown above) that is placed a fixed distance from a wall. Set up your robot as follows:
-
Locate a long, white bubble-wrapped board, either at your table or at the sides of the room. To stand a wall up, either lean it against the side of a lab table or use the attached metal clips. If you are having trouble finding a wall, ask a staff member for help.
-
Find a robot.
-
Attach a LEGO Lance to the front of your robot.
-
Place the bull's eye target about 1 meter in front of the robot. The perpendicular distance from the bull's eye to the bubble wall should be 40 cm.
-
Start the robot at the same initial conditions (0.5 meters from the wall and rotated \pi / 4 radians to the left) that were used in the simulator.
-
Start by placing a business card in the ring, and test whether the robot can hit the card with its lance. If not, fix it (among other things, you may need to adjust the gains from what you found in simulation). Then remove the business card and try to spear the bull's eye. Note that you may need to adjust the height of your LEGO lance in order to spear the target.
Demonstrate hitting the bull's eye. What gain pairs worked best on the real robot? How did this compare against the soar simulation and your LTI model?
Can you hit the bull's eye if it the robot starts just 2 feet away? Can you do it twice in a row?